I present you the Samucs Web DICOM Viewer approach. This is a software designed for DICOM image visualization. Since it's a web based program it runs entirely inside your web browser and also makes use of the power of Ajax and Java technologies that increase performance and portability, enhancing flawlessly the user experience.
It's the result of several days of hard work. Here I post the first version of the application for testing and feedback. In addition this software uses the powerful dcm4che toolkit - a collection of open source applications and utilities for the healthcare enterprise. These applications have been also developed in the Java programming, supporting deployment on JDK 1.4 and up.
Features included in this version:
- Remote AE title setup (PACS communication);
- Perform queries on Study, Series and Instance levels;
- Query by filter;
- Query and retrieve images for visualization (C-GET, C-MOVE);
- DICOM header viewer;
- Tools for zooming and image adjustments;
- Different layout views;
To start viewing images right now, first download Samucs Web DICOM Viewer. Then extract the
samucs-web.rar
to get a WAR file that must be deployed to a Web Server. So download the great Web Server Apache Tomcat. Also, the Java Runtime Environment (JRE) should be running properly on your computer. This application was tested only on Windows Vista environment using Internet Explorer 7 and Firefox 3.0, JRE 6 and Tomcat 6. I strongly recommend Firefox. Linux users I'll try this OS soon :)The Tomcat install is quite intuitive, just follow the instructions and be sure to set port 8080 for Http. In the end you'll be asked to run Tomcat. Then open your web browser and access
http://localhost:8080
. If everything is ok you'll see the Tomcat management page. Then click the link Tomcat Manager
on the top left corner below the Cat and enter the username and password saved during the installation. Now you are able to deploy the Web application to the server. Scroll down the page until you find the box WAR file to deploy
and then select the downloaded WAR file. Finally click the button Deploy
. It may be necessary to restart Tomcat before running the application.That's it! Access
http://localhost:8080/samucs-web
to begin the new DICOM visualization experience. The default username and password is samucs/samucs
. I appreciate your feedback.Screenshots:
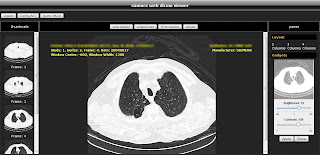
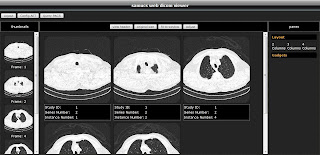
Enjoy :)
Kindly regards,
Samuel.